Sending Email with Amazon SES and SST
A great advantage of using SST is that you can combine various components. Here I’ll explain how you can use Amazon Simple Email Service (SES) to send emails to yourself.
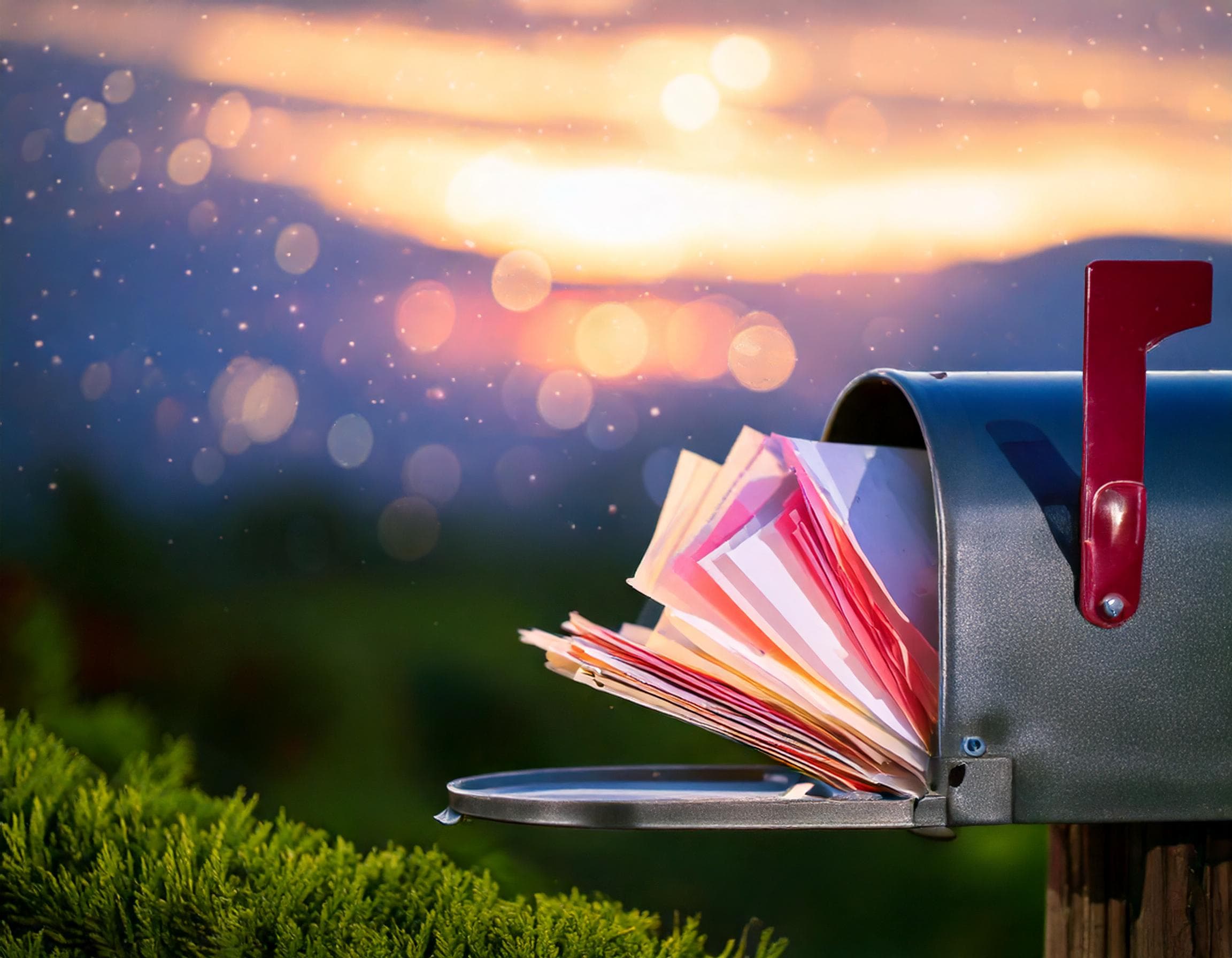
Configuring SST
Configuring of email is simple with SST. Here we have sst.config.ts
again:
// <reference path="./.sst/platform/config.d.ts" />
export default $config({
app(input) {
return {
name: 'sst-nextjs-test',
removal: input?.stage === 'production' ? 'retain' : 'remove',
protect: ['production'].includes(input?.stage),
home: 'aws',
}
},
async run() {
const email = sst.aws.Email.get('MyEmail', 'mail@example.com')
new sst.aws.Nextjs('MyWeb', {
domain:
$app.stage === 'production'
? {
name: 'example.com',
redirects: ['www.example.com'],
}
: undefined,
link: [email],
})
},
})
Now I’ve added the line:
const email = sst.aws.Email.get('MyEmail', 'mail@example.com')
I use Email.get
here because I’ve already created a configuration of Amazon SES at AWS. You can also create a new email identity like this:
const email = new sst.aws.Email('MyEmail', { sender: 'mail@example.com' })
When you’re starting out with Amazon SES you will be working in sandbox mode, and each email address that you use must be verified by clicking on the verification link in the email that is sent to the address. When activating production mode you can configure a domain as sender, and send from any email address using this domain, as long as the domain is configured correctly.
It’s also important to remember to link the email component to the Next.js component. I’ve done this by adding link: [email]
to the configuration object. I forgot to do this in the beginning, and got an error message saying I didn’t have permission to send email. By linking, SST will add the necessary permission.
This linking works for all components of SST. It is a very flexible concept, that can be used to build advanced cloud services.
Sending email
You can send email by using the SDK in the npm package @aws-sdk/client-ses
. Here’s some example code:
import { SendEmailCommand, SESClient } from '@aws-sdk/client-ses'
const sesClient = new SESClient({
region: 'eu-west-1',
})
export async function sendEmail(message: string, email: string) {
const from = 'mail@example.com'
const to = 'mail@example.com'
const sendEmailCommand = new SendEmailCommand({
Destination: {
ToAddresses: [to],
},
Message: {
Body: {
Text: {
Charset: 'UTF-8',
Data: message,
},
},
Subject: {
Charset: 'UTF-8',
Data: `Message from <${email}>`,
},
},
ReplyToAddresses: [email],
Source: from,
})
try {
await sesClient.send(sendEmailCommand)
} catch (error) {
console.error(error)
}
}
I use a similar function as this to send myself an email whenever someone submits the contact form.
If you use this code for a similar purpose, remember to validate any input from the client. For example I have code that validates that the submitted email address is a real email address. I recommend using the package deep-email-validator
for validating email addresses.
Conclusion
As you can see, it is quite easy to configure SST to support sending email through Amazon SES. You can find more information in the documentation for the email component.
This little example is a good illustration of how you can link components together in SST. This is a very powerful tool that can be used to build advanced cloud services with SST.
Hope you found this article useful. Please get in touch if you have questions or comments.